Deal the cards to the playersCreate chunks from an arraySimple One Game BlackjackName the poker hand - 7...
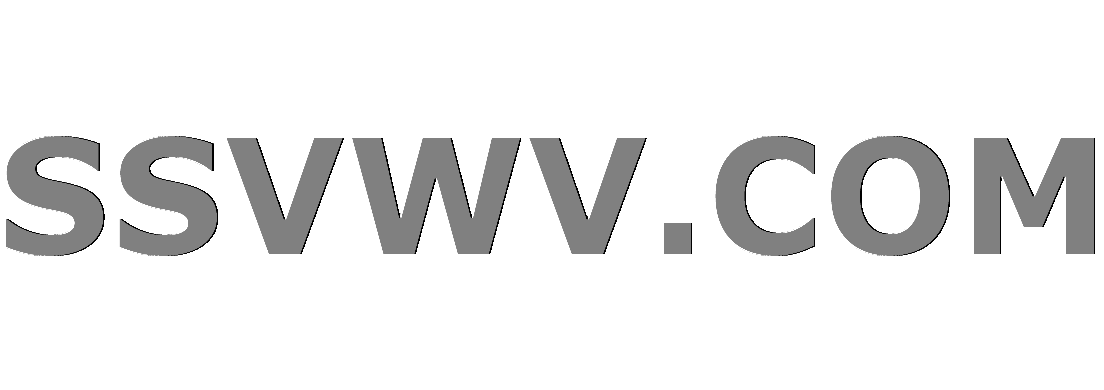
Multi tool use
Quitting employee has privileged access to critical information
How do I enter < and > on a broken keyboard?
How to draw tikz paths composed only of horizontal, vertical and diagonal segments?
How do we objectively assess if a dialogue sounds unnatural or cringy?
How will Occam's Razor principle work in Machine learning
Has Wakanda ever accepted refugees?
Can an earth elemental drown/bury its opponent underground using earth glide?
Is divide-by-zero a security vulnerability?
Can a Tiny Servant be used as a messenger?
How does insurance birth control work?
What's Bob's age again?
Can I solder 12/2 Romex to extend wire 5 ft?
How can I handle a player who pre-plans arguments about my rulings on RAW?
PTIJ: Mordechai mourning
How do you write a macro that takes arguments containing paragraphs?
PTIJ: What dummy is the Gemara referring to?
What is better: yes / no radio, or simple checkbox?
“I had a flat in the centre of town, but I didn’t like living there, so …”
A bug in Excel? Conditional formatting for marking duplicates also highlights unique value
Are Wave equations equivalent to Maxwell equations in free space?
The orphan's family
An Undercover Army
Where does the proton come in the reduction of NAD?
What can I do if someone tampers with my SSH public key?
Deal the cards to the players
Create chunks from an arraySimple One Game BlackjackName the poker hand - 7 cards editionCompare two poker handsGolf me a card dealerScore a hand of Cribbage!1326 starting hold'em combosDeal an ASCII DeckWant to see a Magic Card Trick?Blackjack Bust CalculatorCreate chunks from an array
$begingroup$
Tonight is card game night! You are the dealer and your task is to write a program to deal the cards to the players.
Given an array of cards and the number of players, you need to split the array of cards into a hand for each player.
Rules
Your program will receive an non-empty array A
, as well as a non-zero positive integer n
. The array should then be split into n
hands. If the length of the string isn't divisible by n
any leftover cards at the end should be distributed as evenly as possible.
- If
n==1
, you will need to return an array of array withA
as it's only element If
n
is greater than the length ofA
, you will need to return every hand and an empty hand. ifn = 4
andarray A = [1,2,3]
, you should return[[1],[2],[3]]
or[[1],[2],[3],[]]
. You are free to handle the empty hand with empty, undefined or null.The array can contain any type rather than a number.
You should not change the order of the array while dealing. For example
if n = 2
andA= [1,2,3]
, any result rather than[[1,3],[2]]
will be invalid.
Test Cases
n A Output
1 [1,2,3,4,5,6] [[1,2,3,4,5,6]]
2 [1,2,3,4,5,6] [[1,3,5],[2,4,6]]
3 [1,2,3,4,5,6] [[1,4],[2,5],[3,6]]
4 [1,2,3,4,5,6] [[1,5],[2,6],[3],[4]]
7 [1,2,3,4,5,6] [[1],[2],[3],[4],[5],[6]] // or [[1],[2],[3],[4],[5],[6],[]]
Demo Program
def deal(cards, n):
i = 0
players = [[] for _ in range(n)]
for card in cards:
players[i % n].append(card)
i += 1
return players
hands = deal([1,2,3,4,5,6], 2)
print(hands)
Try it online!
This is code-golf, so you the shortest bytes of each language will be the winner.
Inspired from Create chunks from array by chau giang
code-golf array-manipulation
$endgroup$
|
show 15 more comments
$begingroup$
Tonight is card game night! You are the dealer and your task is to write a program to deal the cards to the players.
Given an array of cards and the number of players, you need to split the array of cards into a hand for each player.
Rules
Your program will receive an non-empty array A
, as well as a non-zero positive integer n
. The array should then be split into n
hands. If the length of the string isn't divisible by n
any leftover cards at the end should be distributed as evenly as possible.
- If
n==1
, you will need to return an array of array withA
as it's only element If
n
is greater than the length ofA
, you will need to return every hand and an empty hand. ifn = 4
andarray A = [1,2,3]
, you should return[[1],[2],[3]]
or[[1],[2],[3],[]]
. You are free to handle the empty hand with empty, undefined or null.The array can contain any type rather than a number.
You should not change the order of the array while dealing. For example
if n = 2
andA= [1,2,3]
, any result rather than[[1,3],[2]]
will be invalid.
Test Cases
n A Output
1 [1,2,3,4,5,6] [[1,2,3,4,5,6]]
2 [1,2,3,4,5,6] [[1,3,5],[2,4,6]]
3 [1,2,3,4,5,6] [[1,4],[2,5],[3,6]]
4 [1,2,3,4,5,6] [[1,5],[2,6],[3],[4]]
7 [1,2,3,4,5,6] [[1],[2],[3],[4],[5],[6]] // or [[1],[2],[3],[4],[5],[6],[]]
Demo Program
def deal(cards, n):
i = 0
players = [[] for _ in range(n)]
for card in cards:
players[i % n].append(card)
i += 1
return players
hands = deal([1,2,3,4,5,6], 2)
print(hands)
Try it online!
This is code-golf, so you the shortest bytes of each language will be the winner.
Inspired from Create chunks from array by chau giang
code-golf array-manipulation
$endgroup$
2
$begingroup$
All the test cases seem to splitA
inton
chunks rather than into chunks of sizen
.
$endgroup$
– Adám
16 hours ago
2
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
15 hours ago
2
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
15 hours ago
2
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
15 hours ago
4
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
15 hours ago
|
show 15 more comments
$begingroup$
Tonight is card game night! You are the dealer and your task is to write a program to deal the cards to the players.
Given an array of cards and the number of players, you need to split the array of cards into a hand for each player.
Rules
Your program will receive an non-empty array A
, as well as a non-zero positive integer n
. The array should then be split into n
hands. If the length of the string isn't divisible by n
any leftover cards at the end should be distributed as evenly as possible.
- If
n==1
, you will need to return an array of array withA
as it's only element If
n
is greater than the length ofA
, you will need to return every hand and an empty hand. ifn = 4
andarray A = [1,2,3]
, you should return[[1],[2],[3]]
or[[1],[2],[3],[]]
. You are free to handle the empty hand with empty, undefined or null.The array can contain any type rather than a number.
You should not change the order of the array while dealing. For example
if n = 2
andA= [1,2,3]
, any result rather than[[1,3],[2]]
will be invalid.
Test Cases
n A Output
1 [1,2,3,4,5,6] [[1,2,3,4,5,6]]
2 [1,2,3,4,5,6] [[1,3,5],[2,4,6]]
3 [1,2,3,4,5,6] [[1,4],[2,5],[3,6]]
4 [1,2,3,4,5,6] [[1,5],[2,6],[3],[4]]
7 [1,2,3,4,5,6] [[1],[2],[3],[4],[5],[6]] // or [[1],[2],[3],[4],[5],[6],[]]
Demo Program
def deal(cards, n):
i = 0
players = [[] for _ in range(n)]
for card in cards:
players[i % n].append(card)
i += 1
return players
hands = deal([1,2,3,4,5,6], 2)
print(hands)
Try it online!
This is code-golf, so you the shortest bytes of each language will be the winner.
Inspired from Create chunks from array by chau giang
code-golf array-manipulation
$endgroup$
Tonight is card game night! You are the dealer and your task is to write a program to deal the cards to the players.
Given an array of cards and the number of players, you need to split the array of cards into a hand for each player.
Rules
Your program will receive an non-empty array A
, as well as a non-zero positive integer n
. The array should then be split into n
hands. If the length of the string isn't divisible by n
any leftover cards at the end should be distributed as evenly as possible.
- If
n==1
, you will need to return an array of array withA
as it's only element If
n
is greater than the length ofA
, you will need to return every hand and an empty hand. ifn = 4
andarray A = [1,2,3]
, you should return[[1],[2],[3]]
or[[1],[2],[3],[]]
. You are free to handle the empty hand with empty, undefined or null.The array can contain any type rather than a number.
You should not change the order of the array while dealing. For example
if n = 2
andA= [1,2,3]
, any result rather than[[1,3],[2]]
will be invalid.
Test Cases
n A Output
1 [1,2,3,4,5,6] [[1,2,3,4,5,6]]
2 [1,2,3,4,5,6] [[1,3,5],[2,4,6]]
3 [1,2,3,4,5,6] [[1,4],[2,5],[3,6]]
4 [1,2,3,4,5,6] [[1,5],[2,6],[3],[4]]
7 [1,2,3,4,5,6] [[1],[2],[3],[4],[5],[6]] // or [[1],[2],[3],[4],[5],[6],[]]
Demo Program
def deal(cards, n):
i = 0
players = [[] for _ in range(n)]
for card in cards:
players[i % n].append(card)
i += 1
return players
hands = deal([1,2,3,4,5,6], 2)
print(hands)
Try it online!
This is code-golf, so you the shortest bytes of each language will be the winner.
Inspired from Create chunks from array by chau giang
code-golf array-manipulation
code-golf array-manipulation
edited 12 hours ago
Embodiment of Ignorance
1,476123
1,476123
asked 16 hours ago
aloisdgaloisdg
1,5671124
1,5671124
2
$begingroup$
All the test cases seem to splitA
inton
chunks rather than into chunks of sizen
.
$endgroup$
– Adám
16 hours ago
2
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
15 hours ago
2
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
15 hours ago
2
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
15 hours ago
4
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
15 hours ago
|
show 15 more comments
2
$begingroup$
All the test cases seem to splitA
inton
chunks rather than into chunks of sizen
.
$endgroup$
– Adám
16 hours ago
2
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
15 hours ago
2
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
15 hours ago
2
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
15 hours ago
4
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
15 hours ago
2
2
$begingroup$
All the test cases seem to split
A
into n
chunks rather than into chunks of size n
.$endgroup$
– Adám
16 hours ago
$begingroup$
All the test cases seem to split
A
into n
chunks rather than into chunks of size n
.$endgroup$
– Adám
16 hours ago
2
2
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
15 hours ago
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
15 hours ago
2
2
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
15 hours ago
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
15 hours ago
2
2
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
15 hours ago
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
15 hours ago
4
4
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
15 hours ago
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
15 hours ago
|
show 15 more comments
16 Answers
16
active
oldest
votes
$begingroup$
R, 46 25 bytes
function(A,n)split(A,1:n)
Try it online!
split
s A
into groups defined by 1:n
, recycling 1:n
until it matches length with A
.
$endgroup$
add a comment |
$begingroup$
Perl 6, 33 24 bytes
->b{*.classify:{$++%b}}
Try it online!
Anonymous curried code block that takes a number and returns a Whatever lambda that takes a list and returns a list of lists. This takes the second option when given a number larger than the length of lists, e.g. f(4)([1,2,3])
returns [[1],[2],[3]]
Explanation:
->b{ } # Anonymous code block that takes a number
* # And returns a Whatever lambda
.classify # That groups by
:{$++%b} # The index modulo the number
$endgroup$
add a comment |
$begingroup$
Wolfram Language (Mathematica), 28 bytes
(s=#;GatherBy[#2,#~Mod~s&])&
Try it online!
$endgroup$
$begingroup$
Very cleverly done!
$endgroup$
– Greg Martin
4 hours ago
add a comment |
$begingroup$
Python 2, 37 bytes
Code:
lambda x,n:[x[i::n]for i in range(n)]
Try it online!
$endgroup$
add a comment |
$begingroup$
05AB1E, 3 1 byte
Saved 2 bytes thanks to Adnan
ι
Try it online!
or as a Test Suite
Explanation
ι # uninterleave
Does exactly what the challenge asks for
$endgroup$
2
$begingroup$
I think this should work as well:ι
$endgroup$
– Adnan
6 hours ago
$begingroup$
@Adnan: Yeah thanks :) Only difference is the empty list forn=7
, but that is an acceptable output format. I've totally missed that built-in :/
$endgroup$
– Emigna
6 hours ago
$begingroup$
So there is a language with a built-in for this! :D
$endgroup$
– aloisdg
3 hours ago
add a comment |
$begingroup$
Japt, 2 bytes
Takes the array as the first input.
óV
Try it
$endgroup$
add a comment |
$begingroup$
J, 13, 11, 10, 9 bytes
(|#)</.]
Try it online!
how (previous explanation, fundamentally the same)
] </.~ (| #)
</.~ NB. box results of grouping
] NB. the right arg by...
| NB. the remainders of dividing...
[ NB. the left arg into...
# NB. the length of each prefix of...
] NB. the right arg,
NB. aka, the integers 1 thru
NB. the length of the right arg
$endgroup$
add a comment |
$begingroup$
Charcoal, 9 bytes
IEθ✂ηιLηθ
Try it online! Link is to verbose version of code. Takes input in the order [n, A]
and outputs each value on its own line and each hand double-spaced from the previous. Explanation:
θ First input `n`
E Map over implicit range
η Second input `A`
✂ Sliced
ι Starting at current index
Lη Ending at length of `A`
θ Taking every `n`th element
I Cast to string
Implicitly print
$endgroup$
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
14 hours ago
add a comment |
$begingroup$
Haskell, 39 bytes
import Data.Lists
(transpose.).chunksOf
Note: Data.Lists
is from the third-party library lists, which is not on Stackage and hence will not appear on Hoogle.
$endgroup$
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meantData.List
, but it doesn't containchunksOf
.
$endgroup$
– Joseph Sible
14 hours ago
$begingroup$
chunksOf
only seems to appear with the signatureInt -> Text -> [Text]
.1
$endgroup$
– Sriotchilism O'Zaic
14 hours ago
$begingroup$
@JosephSible, it's in thelists
package.
$endgroup$
– dfeuer
14 hours ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in thesplit
package and re-exported by thelists
package. There are versions ofchunksOf
for lists, text, sequences, and probably other things.
$endgroup$
– dfeuer
14 hours ago
add a comment |
$begingroup$
JavaScript (Node.js), 53 bytes
A=>n=>g=(i=n)=>i?[...g(--i),A.filter(_=>i--%n==0)]:[]
Try it online!
$endgroup$
add a comment |
$begingroup$
Kotlin, 53 51 49 bytes
{a,n->(0..n-1).map{a.slice(it..a.size-1 step n)}}
The old, incorrect solution only worked for divisors of the array length. I'm certain this can be golfed down.
Try it online!
New contributor
Adam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
invalid
$endgroup$
– ASCII-only
11 hours ago
$begingroup$
Doesn't work whenn
is not a divisor of the length of the list
$endgroup$
– Jo King
11 hours ago
$begingroup$
I see, thanks. Fixing it now
$endgroup$
– Adam
11 hours ago
$begingroup$
I believe this is fixed @ASCII-only
$endgroup$
– Adam
11 hours ago
1
$begingroup$
looks like you can remove the extra pair ot parens
$endgroup$
– ASCII-only
10 hours ago
|
show 6 more comments
$begingroup$
Jelly, 6 2 bytes
sZ
Try it online!
Thanks to @JonathanAllan for saving 4 bytes
$endgroup$
$begingroup$
DoessZ
not work?
$endgroup$
– Jonathan Allan
2 hours ago
$begingroup$
@JonathanAllan yes, somehow missed that. Do you want to post as separate answer or shall I edit mine?
$endgroup$
– Nick Kennedy
53 mins ago
$begingroup$
No you're welcome to edit :)
$endgroup$
– Jonathan Allan
48 mins ago
add a comment |
$begingroup$
APL+WIN 26 or 31 bytes
If individual hands can be represented as columns of a 2D matrix then 26 bytes if an array of arrays then add 5 bytes.
(l,n)⍴((l←⌈(⍴a)÷n)×n←⎕)↑a←⎕
Try it online! ourtesy of Dyalog Classic
or
⊂[1](l,n)⍴((l←⌈(⍴a)÷n)×n←⎕)↑a←⎕
Try it online! Courtesy of Dyalog Classic
Explanation:
a←⎕ prompt for array of cards
((l←⌈(⍴a)÷n)×n←⎕)↑ prompt for integer, pad a with zeros to given even hands
(l,n)⍴ create 2D matrix with each column representing each hand
⊂[1] if required convert to nested vector - APL array of arrays
$endgroup$
add a comment |
$begingroup$
MathGolf, 9 bytes
ô_í%q╞;
Try it online!
Explanation
swap top elements (pops both input onto stack)
ô start block of length 6
_ duplicate TOS (will duplicate the list)
í get total number of iterations of for loop (the other input)
swap top elements
% modulo (picks every n:th item of the list
q print without newline
╞ discard from left of string/array (makes the next player pick cards starting with the next in the deck)
; discard TOS (removes some junk in the end)
$endgroup$
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 43 bytes
a=>b=>{int i=0;return a.GroupBy(_=>i++%b);}
Try it online!
$endgroup$
$begingroup$
@JoKing[1,2,3], 4
should output[[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.
$endgroup$
– aloisdg
16 hours ago
1
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
16 hours ago
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
16 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
16 hours ago
add a comment |
$begingroup$
TSQL, 44 bytes
-- @ : table containing the input
-- column c: value of the card,
-- column a: position on the card in the deck
-- @n : number of players
DECLARE @ table(a int identity(0,1), c varchar(9))
DECLARE @n int = 4
INSERT @ values('1a'),('2c'),('3e'),('4g'),('5i'),('6k')
SELECT string_agg(c,',')FROM @ GROUP BY a%@n
Try it out
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "200"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f181029%2fdeal-the-cards-to-the-players%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
16 Answers
16
active
oldest
votes
16 Answers
16
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
R, 46 25 bytes
function(A,n)split(A,1:n)
Try it online!
split
s A
into groups defined by 1:n
, recycling 1:n
until it matches length with A
.
$endgroup$
add a comment |
$begingroup$
R, 46 25 bytes
function(A,n)split(A,1:n)
Try it online!
split
s A
into groups defined by 1:n
, recycling 1:n
until it matches length with A
.
$endgroup$
add a comment |
$begingroup$
R, 46 25 bytes
function(A,n)split(A,1:n)
Try it online!
split
s A
into groups defined by 1:n
, recycling 1:n
until it matches length with A
.
$endgroup$
R, 46 25 bytes
function(A,n)split(A,1:n)
Try it online!
split
s A
into groups defined by 1:n
, recycling 1:n
until it matches length with A
.
edited 15 hours ago
answered 16 hours ago
GiuseppeGiuseppe
16.7k31052
16.7k31052
add a comment |
add a comment |
$begingroup$
Perl 6, 33 24 bytes
->b{*.classify:{$++%b}}
Try it online!
Anonymous curried code block that takes a number and returns a Whatever lambda that takes a list and returns a list of lists. This takes the second option when given a number larger than the length of lists, e.g. f(4)([1,2,3])
returns [[1],[2],[3]]
Explanation:
->b{ } # Anonymous code block that takes a number
* # And returns a Whatever lambda
.classify # That groups by
:{$++%b} # The index modulo the number
$endgroup$
add a comment |
$begingroup$
Perl 6, 33 24 bytes
->b{*.classify:{$++%b}}
Try it online!
Anonymous curried code block that takes a number and returns a Whatever lambda that takes a list and returns a list of lists. This takes the second option when given a number larger than the length of lists, e.g. f(4)([1,2,3])
returns [[1],[2],[3]]
Explanation:
->b{ } # Anonymous code block that takes a number
* # And returns a Whatever lambda
.classify # That groups by
:{$++%b} # The index modulo the number
$endgroup$
add a comment |
$begingroup$
Perl 6, 33 24 bytes
->b{*.classify:{$++%b}}
Try it online!
Anonymous curried code block that takes a number and returns a Whatever lambda that takes a list and returns a list of lists. This takes the second option when given a number larger than the length of lists, e.g. f(4)([1,2,3])
returns [[1],[2],[3]]
Explanation:
->b{ } # Anonymous code block that takes a number
* # And returns a Whatever lambda
.classify # That groups by
:{$++%b} # The index modulo the number
$endgroup$
Perl 6, 33 24 bytes
->b{*.classify:{$++%b}}
Try it online!
Anonymous curried code block that takes a number and returns a Whatever lambda that takes a list and returns a list of lists. This takes the second option when given a number larger than the length of lists, e.g. f(4)([1,2,3])
returns [[1],[2],[3]]
Explanation:
->b{ } # Anonymous code block that takes a number
* # And returns a Whatever lambda
.classify # That groups by
:{$++%b} # The index modulo the number
edited 15 hours ago
answered 16 hours ago
Jo KingJo King
24.4k357126
24.4k357126
add a comment |
add a comment |
$begingroup$
Wolfram Language (Mathematica), 28 bytes
(s=#;GatherBy[#2,#~Mod~s&])&
Try it online!
$endgroup$
$begingroup$
Very cleverly done!
$endgroup$
– Greg Martin
4 hours ago
add a comment |
$begingroup$
Wolfram Language (Mathematica), 28 bytes
(s=#;GatherBy[#2,#~Mod~s&])&
Try it online!
$endgroup$
$begingroup$
Very cleverly done!
$endgroup$
– Greg Martin
4 hours ago
add a comment |
$begingroup$
Wolfram Language (Mathematica), 28 bytes
(s=#;GatherBy[#2,#~Mod~s&])&
Try it online!
$endgroup$
Wolfram Language (Mathematica), 28 bytes
(s=#;GatherBy[#2,#~Mod~s&])&
Try it online!
answered 15 hours ago


J42161217J42161217
13.2k21150
13.2k21150
$begingroup$
Very cleverly done!
$endgroup$
– Greg Martin
4 hours ago
add a comment |
$begingroup$
Very cleverly done!
$endgroup$
– Greg Martin
4 hours ago
$begingroup$
Very cleverly done!
$endgroup$
– Greg Martin
4 hours ago
$begingroup$
Very cleverly done!
$endgroup$
– Greg Martin
4 hours ago
add a comment |
$begingroup$
Python 2, 37 bytes
Code:
lambda x,n:[x[i::n]for i in range(n)]
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 37 bytes
Code:
lambda x,n:[x[i::n]for i in range(n)]
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 37 bytes
Code:
lambda x,n:[x[i::n]for i in range(n)]
Try it online!
$endgroup$
Python 2, 37 bytes
Code:
lambda x,n:[x[i::n]for i in range(n)]
Try it online!
answered 15 hours ago


AdnanAdnan
35.7k562225
35.7k562225
add a comment |
add a comment |
$begingroup$
05AB1E, 3 1 byte
Saved 2 bytes thanks to Adnan
ι
Try it online!
or as a Test Suite
Explanation
ι # uninterleave
Does exactly what the challenge asks for
$endgroup$
2
$begingroup$
I think this should work as well:ι
$endgroup$
– Adnan
6 hours ago
$begingroup$
@Adnan: Yeah thanks :) Only difference is the empty list forn=7
, but that is an acceptable output format. I've totally missed that built-in :/
$endgroup$
– Emigna
6 hours ago
$begingroup$
So there is a language with a built-in for this! :D
$endgroup$
– aloisdg
3 hours ago
add a comment |
$begingroup$
05AB1E, 3 1 byte
Saved 2 bytes thanks to Adnan
ι
Try it online!
or as a Test Suite
Explanation
ι # uninterleave
Does exactly what the challenge asks for
$endgroup$
2
$begingroup$
I think this should work as well:ι
$endgroup$
– Adnan
6 hours ago
$begingroup$
@Adnan: Yeah thanks :) Only difference is the empty list forn=7
, but that is an acceptable output format. I've totally missed that built-in :/
$endgroup$
– Emigna
6 hours ago
$begingroup$
So there is a language with a built-in for this! :D
$endgroup$
– aloisdg
3 hours ago
add a comment |
$begingroup$
05AB1E, 3 1 byte
Saved 2 bytes thanks to Adnan
ι
Try it online!
or as a Test Suite
Explanation
ι # uninterleave
Does exactly what the challenge asks for
$endgroup$
05AB1E, 3 1 byte
Saved 2 bytes thanks to Adnan
ι
Try it online!
or as a Test Suite
Explanation
ι # uninterleave
Does exactly what the challenge asks for
edited 6 hours ago
answered 8 hours ago


EmignaEmigna
46.8k432142
46.8k432142
2
$begingroup$
I think this should work as well:ι
$endgroup$
– Adnan
6 hours ago
$begingroup$
@Adnan: Yeah thanks :) Only difference is the empty list forn=7
, but that is an acceptable output format. I've totally missed that built-in :/
$endgroup$
– Emigna
6 hours ago
$begingroup$
So there is a language with a built-in for this! :D
$endgroup$
– aloisdg
3 hours ago
add a comment |
2
$begingroup$
I think this should work as well:ι
$endgroup$
– Adnan
6 hours ago
$begingroup$
@Adnan: Yeah thanks :) Only difference is the empty list forn=7
, but that is an acceptable output format. I've totally missed that built-in :/
$endgroup$
– Emigna
6 hours ago
$begingroup$
So there is a language with a built-in for this! :D
$endgroup$
– aloisdg
3 hours ago
2
2
$begingroup$
I think this should work as well:
ι
$endgroup$
– Adnan
6 hours ago
$begingroup$
I think this should work as well:
ι
$endgroup$
– Adnan
6 hours ago
$begingroup$
@Adnan: Yeah thanks :) Only difference is the empty list for
n=7
, but that is an acceptable output format. I've totally missed that built-in :/$endgroup$
– Emigna
6 hours ago
$begingroup$
@Adnan: Yeah thanks :) Only difference is the empty list for
n=7
, but that is an acceptable output format. I've totally missed that built-in :/$endgroup$
– Emigna
6 hours ago
$begingroup$
So there is a language with a built-in for this! :D
$endgroup$
– aloisdg
3 hours ago
$begingroup$
So there is a language with a built-in for this! :D
$endgroup$
– aloisdg
3 hours ago
add a comment |
$begingroup$
Japt, 2 bytes
Takes the array as the first input.
óV
Try it
$endgroup$
add a comment |
$begingroup$
Japt, 2 bytes
Takes the array as the first input.
óV
Try it
$endgroup$
add a comment |
$begingroup$
Japt, 2 bytes
Takes the array as the first input.
óV
Try it
$endgroup$
Japt, 2 bytes
Takes the array as the first input.
óV
Try it
answered 16 hours ago


ShaggyShaggy
19.4k21667
19.4k21667
add a comment |
add a comment |
$begingroup$
J, 13, 11, 10, 9 bytes
(|#)</.]
Try it online!
how (previous explanation, fundamentally the same)
] </.~ (| #)
</.~ NB. box results of grouping
] NB. the right arg by...
| NB. the remainders of dividing...
[ NB. the left arg into...
# NB. the length of each prefix of...
] NB. the right arg,
NB. aka, the integers 1 thru
NB. the length of the right arg
$endgroup$
add a comment |
$begingroup$
J, 13, 11, 10, 9 bytes
(|#)</.]
Try it online!
how (previous explanation, fundamentally the same)
] </.~ (| #)
</.~ NB. box results of grouping
] NB. the right arg by...
| NB. the remainders of dividing...
[ NB. the left arg into...
# NB. the length of each prefix of...
] NB. the right arg,
NB. aka, the integers 1 thru
NB. the length of the right arg
$endgroup$
add a comment |
$begingroup$
J, 13, 11, 10, 9 bytes
(|#)</.]
Try it online!
how (previous explanation, fundamentally the same)
] </.~ (| #)
</.~ NB. box results of grouping
] NB. the right arg by...
| NB. the remainders of dividing...
[ NB. the left arg into...
# NB. the length of each prefix of...
] NB. the right arg,
NB. aka, the integers 1 thru
NB. the length of the right arg
$endgroup$
J, 13, 11, 10, 9 bytes
(|#)</.]
Try it online!
how (previous explanation, fundamentally the same)
] </.~ (| #)
</.~ NB. box results of grouping
] NB. the right arg by...
| NB. the remainders of dividing...
[ NB. the left arg into...
# NB. the length of each prefix of...
] NB. the right arg,
NB. aka, the integers 1 thru
NB. the length of the right arg
edited 13 hours ago
answered 14 hours ago


JonahJonah
2,4511017
2,4511017
add a comment |
add a comment |
$begingroup$
Charcoal, 9 bytes
IEθ✂ηιLηθ
Try it online! Link is to verbose version of code. Takes input in the order [n, A]
and outputs each value on its own line and each hand double-spaced from the previous. Explanation:
θ First input `n`
E Map over implicit range
η Second input `A`
✂ Sliced
ι Starting at current index
Lη Ending at length of `A`
θ Taking every `n`th element
I Cast to string
Implicitly print
$endgroup$
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
14 hours ago
add a comment |
$begingroup$
Charcoal, 9 bytes
IEθ✂ηιLηθ
Try it online! Link is to verbose version of code. Takes input in the order [n, A]
and outputs each value on its own line and each hand double-spaced from the previous. Explanation:
θ First input `n`
E Map over implicit range
η Second input `A`
✂ Sliced
ι Starting at current index
Lη Ending at length of `A`
θ Taking every `n`th element
I Cast to string
Implicitly print
$endgroup$
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
14 hours ago
add a comment |
$begingroup$
Charcoal, 9 bytes
IEθ✂ηιLηθ
Try it online! Link is to verbose version of code. Takes input in the order [n, A]
and outputs each value on its own line and each hand double-spaced from the previous. Explanation:
θ First input `n`
E Map over implicit range
η Second input `A`
✂ Sliced
ι Starting at current index
Lη Ending at length of `A`
θ Taking every `n`th element
I Cast to string
Implicitly print
$endgroup$
Charcoal, 9 bytes
IEθ✂ηιLηθ
Try it online! Link is to verbose version of code. Takes input in the order [n, A]
and outputs each value on its own line and each hand double-spaced from the previous. Explanation:
θ First input `n`
E Map over implicit range
η Second input `A`
✂ Sliced
ι Starting at current index
Lη Ending at length of `A`
θ Taking every `n`th element
I Cast to string
Implicitly print
answered 15 hours ago
NeilNeil
81.4k745178
81.4k745178
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
14 hours ago
add a comment |
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
14 hours ago
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
14 hours ago
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
14 hours ago
add a comment |
$begingroup$
Haskell, 39 bytes
import Data.Lists
(transpose.).chunksOf
Note: Data.Lists
is from the third-party library lists, which is not on Stackage and hence will not appear on Hoogle.
$endgroup$
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meantData.List
, but it doesn't containchunksOf
.
$endgroup$
– Joseph Sible
14 hours ago
$begingroup$
chunksOf
only seems to appear with the signatureInt -> Text -> [Text]
.1
$endgroup$
– Sriotchilism O'Zaic
14 hours ago
$begingroup$
@JosephSible, it's in thelists
package.
$endgroup$
– dfeuer
14 hours ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in thesplit
package and re-exported by thelists
package. There are versions ofchunksOf
for lists, text, sequences, and probably other things.
$endgroup$
– dfeuer
14 hours ago
add a comment |
$begingroup$
Haskell, 39 bytes
import Data.Lists
(transpose.).chunksOf
Note: Data.Lists
is from the third-party library lists, which is not on Stackage and hence will not appear on Hoogle.
$endgroup$
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meantData.List
, but it doesn't containchunksOf
.
$endgroup$
– Joseph Sible
14 hours ago
$begingroup$
chunksOf
only seems to appear with the signatureInt -> Text -> [Text]
.1
$endgroup$
– Sriotchilism O'Zaic
14 hours ago
$begingroup$
@JosephSible, it's in thelists
package.
$endgroup$
– dfeuer
14 hours ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in thesplit
package and re-exported by thelists
package. There are versions ofchunksOf
for lists, text, sequences, and probably other things.
$endgroup$
– dfeuer
14 hours ago
add a comment |
$begingroup$
Haskell, 39 bytes
import Data.Lists
(transpose.).chunksOf
Note: Data.Lists
is from the third-party library lists, which is not on Stackage and hence will not appear on Hoogle.
$endgroup$
Haskell, 39 bytes
import Data.Lists
(transpose.).chunksOf
Note: Data.Lists
is from the third-party library lists, which is not on Stackage and hence will not appear on Hoogle.
edited 14 hours ago


Joseph Sible
2075
2075
answered 15 hours ago


dfeuerdfeuer
44138
44138
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meantData.List
, but it doesn't containchunksOf
.
$endgroup$
– Joseph Sible
14 hours ago
$begingroup$
chunksOf
only seems to appear with the signatureInt -> Text -> [Text]
.1
$endgroup$
– Sriotchilism O'Zaic
14 hours ago
$begingroup$
@JosephSible, it's in thelists
package.
$endgroup$
– dfeuer
14 hours ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in thesplit
package and re-exported by thelists
package. There are versions ofchunksOf
for lists, text, sequences, and probably other things.
$endgroup$
– dfeuer
14 hours ago
add a comment |
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meantData.List
, but it doesn't containchunksOf
.
$endgroup$
– Joseph Sible
14 hours ago
$begingroup$
chunksOf
only seems to appear with the signatureInt -> Text -> [Text]
.1
$endgroup$
– Sriotchilism O'Zaic
14 hours ago
$begingroup$
@JosephSible, it's in thelists
package.
$endgroup$
– dfeuer
14 hours ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in thesplit
package and re-exported by thelists
package. There are versions ofchunksOf
for lists, text, sequences, and probably other things.
$endgroup$
– dfeuer
14 hours ago
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meant Data.List
, but it doesn't contain chunksOf
.$endgroup$
– Joseph Sible
14 hours ago
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meant Data.List
, but it doesn't contain chunksOf
.$endgroup$
– Joseph Sible
14 hours ago
$begingroup$
chunksOf
only seems to appear with the signature Int -> Text -> [Text]
.1$endgroup$
– Sriotchilism O'Zaic
14 hours ago
$begingroup$
chunksOf
only seems to appear with the signature Int -> Text -> [Text]
.1$endgroup$
– Sriotchilism O'Zaic
14 hours ago
$begingroup$
@JosephSible, it's in the
lists
package.$endgroup$
– dfeuer
14 hours ago
$begingroup$
@JosephSible, it's in the
lists
package.$endgroup$
– dfeuer
14 hours ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in the
split
package and re-exported by the lists
package. There are versions of chunksOf
for lists, text, sequences, and probably other things.$endgroup$
– dfeuer
14 hours ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in the
split
package and re-exported by the lists
package. There are versions of chunksOf
for lists, text, sequences, and probably other things.$endgroup$
– dfeuer
14 hours ago
add a comment |
$begingroup$
JavaScript (Node.js), 53 bytes
A=>n=>g=(i=n)=>i?[...g(--i),A.filter(_=>i--%n==0)]:[]
Try it online!
$endgroup$
add a comment |
$begingroup$
JavaScript (Node.js), 53 bytes
A=>n=>g=(i=n)=>i?[...g(--i),A.filter(_=>i--%n==0)]:[]
Try it online!
$endgroup$
add a comment |
$begingroup$
JavaScript (Node.js), 53 bytes
A=>n=>g=(i=n)=>i?[...g(--i),A.filter(_=>i--%n==0)]:[]
Try it online!
$endgroup$
JavaScript (Node.js), 53 bytes
A=>n=>g=(i=n)=>i?[...g(--i),A.filter(_=>i--%n==0)]:[]
Try it online!
answered 11 hours ago
l4m2l4m2
4,7861835
4,7861835
add a comment |
add a comment |
$begingroup$
Kotlin, 53 51 49 bytes
{a,n->(0..n-1).map{a.slice(it..a.size-1 step n)}}
The old, incorrect solution only worked for divisors of the array length. I'm certain this can be golfed down.
Try it online!
New contributor
Adam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
invalid
$endgroup$
– ASCII-only
11 hours ago
$begingroup$
Doesn't work whenn
is not a divisor of the length of the list
$endgroup$
– Jo King
11 hours ago
$begingroup$
I see, thanks. Fixing it now
$endgroup$
– Adam
11 hours ago
$begingroup$
I believe this is fixed @ASCII-only
$endgroup$
– Adam
11 hours ago
1
$begingroup$
looks like you can remove the extra pair ot parens
$endgroup$
– ASCII-only
10 hours ago
|
show 6 more comments
$begingroup$
Kotlin, 53 51 49 bytes
{a,n->(0..n-1).map{a.slice(it..a.size-1 step n)}}
The old, incorrect solution only worked for divisors of the array length. I'm certain this can be golfed down.
Try it online!
New contributor
Adam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
invalid
$endgroup$
– ASCII-only
11 hours ago
$begingroup$
Doesn't work whenn
is not a divisor of the length of the list
$endgroup$
– Jo King
11 hours ago
$begingroup$
I see, thanks. Fixing it now
$endgroup$
– Adam
11 hours ago
$begingroup$
I believe this is fixed @ASCII-only
$endgroup$
– Adam
11 hours ago
1
$begingroup$
looks like you can remove the extra pair ot parens
$endgroup$
– ASCII-only
10 hours ago
|
show 6 more comments
$begingroup$
Kotlin, 53 51 49 bytes
{a,n->(0..n-1).map{a.slice(it..a.size-1 step n)}}
The old, incorrect solution only worked for divisors of the array length. I'm certain this can be golfed down.
Try it online!
New contributor
Adam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
Kotlin, 53 51 49 bytes
{a,n->(0..n-1).map{a.slice(it..a.size-1 step n)}}
The old, incorrect solution only worked for divisors of the array length. I'm certain this can be golfed down.
Try it online!
New contributor
Adam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 10 hours ago
New contributor
Adam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 11 hours ago


AdamAdam
414
414
New contributor
Adam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Adam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Adam is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$begingroup$
invalid
$endgroup$
– ASCII-only
11 hours ago
$begingroup$
Doesn't work whenn
is not a divisor of the length of the list
$endgroup$
– Jo King
11 hours ago
$begingroup$
I see, thanks. Fixing it now
$endgroup$
– Adam
11 hours ago
$begingroup$
I believe this is fixed @ASCII-only
$endgroup$
– Adam
11 hours ago
1
$begingroup$
looks like you can remove the extra pair ot parens
$endgroup$
– ASCII-only
10 hours ago
|
show 6 more comments
$begingroup$
invalid
$endgroup$
– ASCII-only
11 hours ago
$begingroup$
Doesn't work whenn
is not a divisor of the length of the list
$endgroup$
– Jo King
11 hours ago
$begingroup$
I see, thanks. Fixing it now
$endgroup$
– Adam
11 hours ago
$begingroup$
I believe this is fixed @ASCII-only
$endgroup$
– Adam
11 hours ago
1
$begingroup$
looks like you can remove the extra pair ot parens
$endgroup$
– ASCII-only
10 hours ago
$begingroup$
invalid
$endgroup$
– ASCII-only
11 hours ago
$begingroup$
invalid
$endgroup$
– ASCII-only
11 hours ago
$begingroup$
Doesn't work when
n
is not a divisor of the length of the list$endgroup$
– Jo King
11 hours ago
$begingroup$
Doesn't work when
n
is not a divisor of the length of the list$endgroup$
– Jo King
11 hours ago
$begingroup$
I see, thanks. Fixing it now
$endgroup$
– Adam
11 hours ago
$begingroup$
I see, thanks. Fixing it now
$endgroup$
– Adam
11 hours ago
$begingroup$
I believe this is fixed @ASCII-only
$endgroup$
– Adam
11 hours ago
$begingroup$
I believe this is fixed @ASCII-only
$endgroup$
– Adam
11 hours ago
1
1
$begingroup$
looks like you can remove the extra pair ot parens
$endgroup$
– ASCII-only
10 hours ago
$begingroup$
looks like you can remove the extra pair ot parens
$endgroup$
– ASCII-only
10 hours ago
|
show 6 more comments
$begingroup$
Jelly, 6 2 bytes
sZ
Try it online!
Thanks to @JonathanAllan for saving 4 bytes
$endgroup$
$begingroup$
DoessZ
not work?
$endgroup$
– Jonathan Allan
2 hours ago
$begingroup$
@JonathanAllan yes, somehow missed that. Do you want to post as separate answer or shall I edit mine?
$endgroup$
– Nick Kennedy
53 mins ago
$begingroup$
No you're welcome to edit :)
$endgroup$
– Jonathan Allan
48 mins ago
add a comment |
$begingroup$
Jelly, 6 2 bytes
sZ
Try it online!
Thanks to @JonathanAllan for saving 4 bytes
$endgroup$
$begingroup$
DoessZ
not work?
$endgroup$
– Jonathan Allan
2 hours ago
$begingroup$
@JonathanAllan yes, somehow missed that. Do you want to post as separate answer or shall I edit mine?
$endgroup$
– Nick Kennedy
53 mins ago
$begingroup$
No you're welcome to edit :)
$endgroup$
– Jonathan Allan
48 mins ago
add a comment |
$begingroup$
Jelly, 6 2 bytes
sZ
Try it online!
Thanks to @JonathanAllan for saving 4 bytes
$endgroup$
Jelly, 6 2 bytes
sZ
Try it online!
Thanks to @JonathanAllan for saving 4 bytes
edited 36 mins ago
answered 14 hours ago
Nick KennedyNick Kennedy
49127
49127
$begingroup$
DoessZ
not work?
$endgroup$
– Jonathan Allan
2 hours ago
$begingroup$
@JonathanAllan yes, somehow missed that. Do you want to post as separate answer or shall I edit mine?
$endgroup$
– Nick Kennedy
53 mins ago
$begingroup$
No you're welcome to edit :)
$endgroup$
– Jonathan Allan
48 mins ago
add a comment |
$begingroup$
DoessZ
not work?
$endgroup$
– Jonathan Allan
2 hours ago
$begingroup$
@JonathanAllan yes, somehow missed that. Do you want to post as separate answer or shall I edit mine?
$endgroup$
– Nick Kennedy
53 mins ago
$begingroup$
No you're welcome to edit :)
$endgroup$
– Jonathan Allan
48 mins ago
$begingroup$
Does
sZ
not work?$endgroup$
– Jonathan Allan
2 hours ago
$begingroup$
Does
sZ
not work?$endgroup$
– Jonathan Allan
2 hours ago
$begingroup$
@JonathanAllan yes, somehow missed that. Do you want to post as separate answer or shall I edit mine?
$endgroup$
– Nick Kennedy
53 mins ago
$begingroup$
@JonathanAllan yes, somehow missed that. Do you want to post as separate answer or shall I edit mine?
$endgroup$
– Nick Kennedy
53 mins ago
$begingroup$
No you're welcome to edit :)
$endgroup$
– Jonathan Allan
48 mins ago
$begingroup$
No you're welcome to edit :)
$endgroup$
– Jonathan Allan
48 mins ago
add a comment |
$begingroup$
APL+WIN 26 or 31 bytes
If individual hands can be represented as columns of a 2D matrix then 26 bytes if an array of arrays then add 5 bytes.
(l,n)⍴((l←⌈(⍴a)÷n)×n←⎕)↑a←⎕
Try it online! ourtesy of Dyalog Classic
or
⊂[1](l,n)⍴((l←⌈(⍴a)÷n)×n←⎕)↑a←⎕
Try it online! Courtesy of Dyalog Classic
Explanation:
a←⎕ prompt for array of cards
((l←⌈(⍴a)÷n)×n←⎕)↑ prompt for integer, pad a with zeros to given even hands
(l,n)⍴ create 2D matrix with each column representing each hand
⊂[1] if required convert to nested vector - APL array of arrays
$endgroup$
add a comment |
$begingroup$
APL+WIN 26 or 31 bytes
If individual hands can be represented as columns of a 2D matrix then 26 bytes if an array of arrays then add 5 bytes.
(l,n)⍴((l←⌈(⍴a)÷n)×n←⎕)↑a←⎕
Try it online! ourtesy of Dyalog Classic
or
⊂[1](l,n)⍴((l←⌈(⍴a)÷n)×n←⎕)↑a←⎕
Try it online! Courtesy of Dyalog Classic
Explanation:
a←⎕ prompt for array of cards
((l←⌈(⍴a)÷n)×n←⎕)↑ prompt for integer, pad a with zeros to given even hands
(l,n)⍴ create 2D matrix with each column representing each hand
⊂[1] if required convert to nested vector - APL array of arrays
$endgroup$
add a comment |
$begingroup$
APL+WIN 26 or 31 bytes
If individual hands can be represented as columns of a 2D matrix then 26 bytes if an array of arrays then add 5 bytes.
(l,n)⍴((l←⌈(⍴a)÷n)×n←⎕)↑a←⎕
Try it online! ourtesy of Dyalog Classic
or
⊂[1](l,n)⍴((l←⌈(⍴a)÷n)×n←⎕)↑a←⎕
Try it online! Courtesy of Dyalog Classic
Explanation:
a←⎕ prompt for array of cards
((l←⌈(⍴a)÷n)×n←⎕)↑ prompt for integer, pad a with zeros to given even hands
(l,n)⍴ create 2D matrix with each column representing each hand
⊂[1] if required convert to nested vector - APL array of arrays
$endgroup$
APL+WIN 26 or 31 bytes
If individual hands can be represented as columns of a 2D matrix then 26 bytes if an array of arrays then add 5 bytes.
(l,n)⍴((l←⌈(⍴a)÷n)×n←⎕)↑a←⎕
Try it online! ourtesy of Dyalog Classic
or
⊂[1](l,n)⍴((l←⌈(⍴a)÷n)×n←⎕)↑a←⎕
Try it online! Courtesy of Dyalog Classic
Explanation:
a←⎕ prompt for array of cards
((l←⌈(⍴a)÷n)×n←⎕)↑ prompt for integer, pad a with zeros to given even hands
(l,n)⍴ create 2D matrix with each column representing each hand
⊂[1] if required convert to nested vector - APL array of arrays
edited 7 hours ago
answered 8 hours ago
GrahamGraham
2,50678
2,50678
add a comment |
add a comment |
$begingroup$
MathGolf, 9 bytes
ô_í%q╞;
Try it online!
Explanation
swap top elements (pops both input onto stack)
ô start block of length 6
_ duplicate TOS (will duplicate the list)
í get total number of iterations of for loop (the other input)
swap top elements
% modulo (picks every n:th item of the list
q print without newline
╞ discard from left of string/array (makes the next player pick cards starting with the next in the deck)
; discard TOS (removes some junk in the end)
$endgroup$
add a comment |
$begingroup$
MathGolf, 9 bytes
ô_í%q╞;
Try it online!
Explanation
swap top elements (pops both input onto stack)
ô start block of length 6
_ duplicate TOS (will duplicate the list)
í get total number of iterations of for loop (the other input)
swap top elements
% modulo (picks every n:th item of the list
q print without newline
╞ discard from left of string/array (makes the next player pick cards starting with the next in the deck)
; discard TOS (removes some junk in the end)
$endgroup$
add a comment |
$begingroup$
MathGolf, 9 bytes
ô_í%q╞;
Try it online!
Explanation
swap top elements (pops both input onto stack)
ô start block of length 6
_ duplicate TOS (will duplicate the list)
í get total number of iterations of for loop (the other input)
swap top elements
% modulo (picks every n:th item of the list
q print without newline
╞ discard from left of string/array (makes the next player pick cards starting with the next in the deck)
; discard TOS (removes some junk in the end)
$endgroup$
MathGolf, 9 bytes
ô_í%q╞;
Try it online!
Explanation
swap top elements (pops both input onto stack)
ô start block of length 6
_ duplicate TOS (will duplicate the list)
í get total number of iterations of for loop (the other input)
swap top elements
% modulo (picks every n:th item of the list
q print without newline
╞ discard from left of string/array (makes the next player pick cards starting with the next in the deck)
; discard TOS (removes some junk in the end)
answered 1 hour ago


maxbmaxb
3,15811132
3,15811132
add a comment |
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 43 bytes
a=>b=>{int i=0;return a.GroupBy(_=>i++%b);}
Try it online!
$endgroup$
$begingroup$
@JoKing[1,2,3], 4
should output[[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.
$endgroup$
– aloisdg
16 hours ago
1
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
16 hours ago
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
16 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
16 hours ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 43 bytes
a=>b=>{int i=0;return a.GroupBy(_=>i++%b);}
Try it online!
$endgroup$
$begingroup$
@JoKing[1,2,3], 4
should output[[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.
$endgroup$
– aloisdg
16 hours ago
1
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
16 hours ago
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
16 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
16 hours ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 43 bytes
a=>b=>{int i=0;return a.GroupBy(_=>i++%b);}
Try it online!
$endgroup$
C# (Visual C# Interactive Compiler), 43 bytes
a=>b=>{int i=0;return a.GroupBy(_=>i++%b);}
Try it online!
answered 16 hours ago
aloisdgaloisdg
1,5671124
1,5671124
$begingroup$
@JoKing[1,2,3], 4
should output[[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.
$endgroup$
– aloisdg
16 hours ago
1
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
16 hours ago
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
16 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
16 hours ago
add a comment |
$begingroup$
@JoKing[1,2,3], 4
should output[[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.
$endgroup$
– aloisdg
16 hours ago
1
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
16 hours ago
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
16 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
16 hours ago
$begingroup$
@JoKing
[1,2,3], 4
should output [[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.$endgroup$
– aloisdg
16 hours ago
$begingroup$
@JoKing
[1,2,3], 4
should output [[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.$endgroup$
– aloisdg
16 hours ago
1
1
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
16 hours ago
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
16 hours ago
1
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
16 hours ago
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
16 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
16 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
16 hours ago
add a comment |
$begingroup$
TSQL, 44 bytes
-- @ : table containing the input
-- column c: value of the card,
-- column a: position on the card in the deck
-- @n : number of players
DECLARE @ table(a int identity(0,1), c varchar(9))
DECLARE @n int = 4
INSERT @ values('1a'),('2c'),('3e'),('4g'),('5i'),('6k')
SELECT string_agg(c,',')FROM @ GROUP BY a%@n
Try it out
$endgroup$
add a comment |
$begingroup$
TSQL, 44 bytes
-- @ : table containing the input
-- column c: value of the card,
-- column a: position on the card in the deck
-- @n : number of players
DECLARE @ table(a int identity(0,1), c varchar(9))
DECLARE @n int = 4
INSERT @ values('1a'),('2c'),('3e'),('4g'),('5i'),('6k')
SELECT string_agg(c,',')FROM @ GROUP BY a%@n
Try it out
$endgroup$
add a comment |
$begingroup$
TSQL, 44 bytes
-- @ : table containing the input
-- column c: value of the card,
-- column a: position on the card in the deck
-- @n : number of players
DECLARE @ table(a int identity(0,1), c varchar(9))
DECLARE @n int = 4
INSERT @ values('1a'),('2c'),('3e'),('4g'),('5i'),('6k')
SELECT string_agg(c,',')FROM @ GROUP BY a%@n
Try it out
$endgroup$
TSQL, 44 bytes
-- @ : table containing the input
-- column c: value of the card,
-- column a: position on the card in the deck
-- @n : number of players
DECLARE @ table(a int identity(0,1), c varchar(9))
DECLARE @n int = 4
INSERT @ values('1a'),('2c'),('3e'),('4g'),('5i'),('6k')
SELECT string_agg(c,',')FROM @ GROUP BY a%@n
Try it out
answered 4 hours ago


t-clausen.dkt-clausen.dk
1,884314
1,884314
add a comment |
add a comment |
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f181029%2fdeal-the-cards-to-the-players%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Xy Jz,o5DdD2D00H6PS
2
$begingroup$
All the test cases seem to split
A
inton
chunks rather than into chunks of sizen
.$endgroup$
– Adám
16 hours ago
2
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
15 hours ago
2
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
15 hours ago
2
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
15 hours ago
4
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
15 hours ago